Overview
Hangfire is an open-source framework that helps you to create, process and manage your background jobs, i.e. operations you don't want to put in your request processing pipeline:
- mass notifications/newsletter;
- batch import from xml, csv, json;
- creation of archives;
- firing off web hooks;
- deleting users;
- building different graphs;
- image/video processing;
- purge temporary files;
- recurring automated reports;
- database maintenance.
Many Types of Background Jobs
Hangfire supports several kinds of background tasks – short-running and long-running, CPU intensive and I/O intensive, one shot and recurrent. You don’t need to reinvent the wheel – it is ready to use.
Fire-and-forget
These jobs are executed only once and almost immediately after they are fired.
var jobId = BackgroundJob.Enqueue( () => Console.WriteLine("Fire-and-forget!"));
Delayed
Delayed jobs are executed only once too, but not immediately – only after the specified time interval.
var jobId = BackgroundJob.Schedule( () => Console.WriteLine("Delayed!"), TimeSpan.FromDays(7));
Recurring
Recurring jobs are fired many times on the specified CRON schedule.
RecurringJob.AddOrUpdate( "myrecurringjob", () => Console.WriteLine("Recurring!"), Cron.Daily);
Continuations
Continuations are executed when parent job has finished.
BackgroundJob.ContinueJobWith( jobId, () => Console.WriteLine("Continuation!"));
Batches
Batch is a group of background jobs created atomically.
var batchId = Batch.StartNew(x =>
{
x.Enqueue(() => Console.WriteLine("Job 1"));
x.Enqueue(() => Console.WriteLine("Job 2"));
});
Batch Continuations
Batch continuation is fired after all background jobs in a parent batch have finished.
Batch.ContinueBatchWith(batchId, x =>
{
x.Enqueue(() => Console.WriteLine("Last Job"));
});
Background Process
Use them when you need to run background processes continuously throughout the lifetime of your application.
public class CleanTempDirectoryProcess : IBackgroundProcess
{
public void Execute(BackgroundProcessContext context)
{
Directory.CleanUp(Directory.GetTempDirectory());
context.Wait(TimeSpan.FromHours(1));
}
}
Backed by Persistent Storage
Background jobs are very important part of an application and Hangfire ensures that any job is performed at least once. To persist background job information between application restarts, all the information is saved in your favorite persistent storage. Currently the following storages are officially supported:
- SQL Server
- Redis
- In-Memory (preview)
And support for a lot of other storages are implemented by the community, please see the Extensions page for details.
Storage subsystem is abstracted enough to support RDBMS and NoSQL solutions. If your favorite transactional database system is not supported yet, it’s likely possible to implement its support as an extension.
Automatic Retries
If your background job encounters a problem during its execution, it will be retried automatically after some delay. Hangfire successfully deals with the following problems:
- Exceptions
- Application shutdowns
- Unexpected process terminations
You are also able to retry any background job manually through the programming code or the Dashboard UI:
var jobId = BackgroundJob.Enqueue( () => Console.WriteLine("Hello")); var succeeded = BackgroundJob.Requeue(jobId);
Scale as You Grow
You are not required to make any architecture decisions to start using Hangfire. You can begin with simple setup, where background processing is implemented on the web application side.
Later, when you face performance problems, you can separate the processing among different processes or servers – Hangfire uses distributed locks to handle synchronization issues.
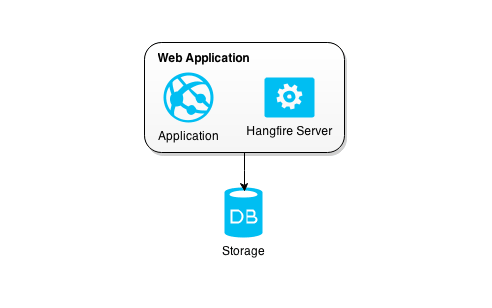
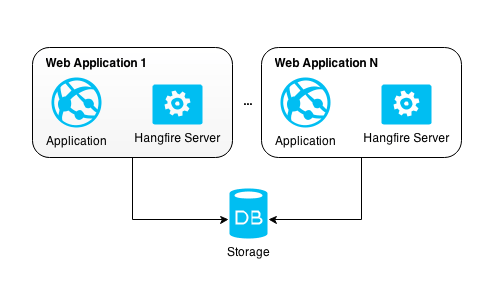
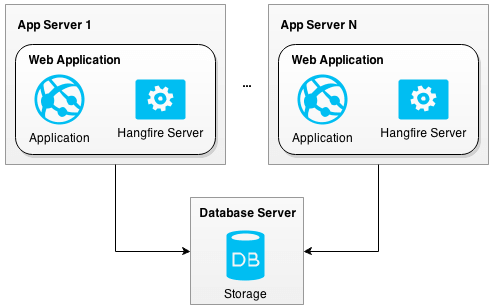
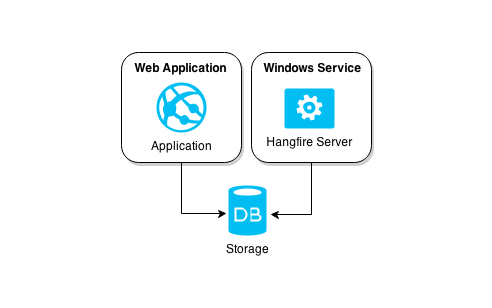
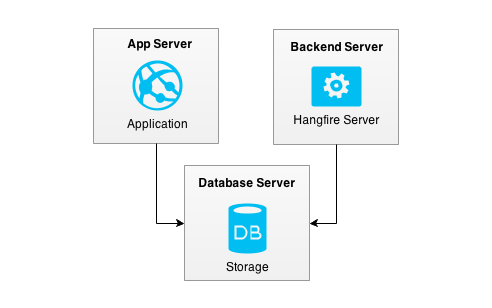
Integrated Monitoring UI
Hangfire is shipped with an awesome tool – Web Monitoring UI. It is implemented as an OWIN extension and can be hosted inside any application – ASP.NET, Console or Windows Service. Monitoring UI allows you to see and control any aspect of background job processing, including statistics, exceptions and background job history.
Just look at the screenshots below, and you’ll love it!